And bypass CORS restrictions at the same time!
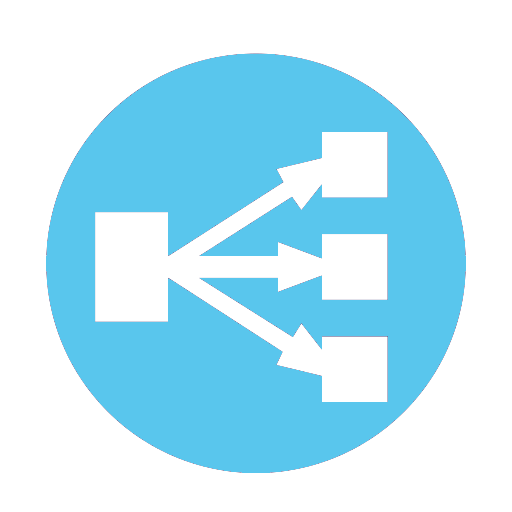
If you need to use a HTTP Proxy with Ajax, this polyfill library will allow you to specify a HTTP proxy to be used with Ajax requests. This could be useful to ensure that your Ajax requests come from a fixed IP address.
Add the library AjaxProxy.js from the following URL
<script src="https://www.ajaxproxy.com/js/ajaxproxy.js"></script>
Before any Ajax requests are made, call:
ajaxProxy.init();
Define your Proxy server as follows;
ajaxProxy.proxy.url = "http://your proxy"; ajaxProxy.proxy.credentials.username = "proxy username"; ajaxProxy.proxy.credentials.password = "proxy password";
If you are using JQuery, then modify your $.Ajax to add headers: ajaxProxy.proxyHeaders() Such as like follows;
$.ajax({ type: "GET", url: "https://ICANHAZIP.COM", headers: ajaxProxy.proxyHeaders(), dataType: "text" }).done (function (data) { console.log(data); });
If you are using plain XHR requests, then add xhr.addProxyHeaders(); Such as shown below;
var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function() { if (this.readyState === 4 && this.status === 200) { console.log(this.responseText); } }; xhr.open("GET", "https://ICANHAZIP.COM", true); xhr.addProxyHeaders(); xhr.send();
If you run your code, then the request should be proxied through your proxy server.
What is happening? In effect, this goes through two levels of proxies, first your request is sent to an AWS Lambda function, which checks for the following http request headers;
X-target : The destination URL X-proxy : The proxy HTTP Address X-proxy-username: The proxy username X-proxy-password: The proxy password
Please note that if your proxy is limited by IP address, then this technique will not work, since the egress IP for the AWS Lambda function is dynamic. You will need a proxy that is either open (not advised), or restricted by username and password. Ajax requests are limited to 3 seconds in duration.
The AWS Lambda function will then make a connection to your proxy server, and supply it with the original destination URL, and will pass through other common headers such as the Content-Type and Authorization headers.
Using the technique above, your proxy username and password will be visible to anyone who can view the source of your website. If you have an intranet, and trust your users, then this may be fine, however, we do recommend taking the following security step;
You can encrypt your proxy username and password by calling:
ajaxProxy.Encrypt("password");
Which will return a string such as follows
:ENCRYPTED:H20n0hTqmOduBYNOatwApO1almPAr/ue
You can pass this string in place of the username and/or password and the script will do the decryption under the hood. There is no public method to reverse this encryption, so it is not possible for an attacker to reverse engineer your password without stealing our private keys, which we keep secret.
If you would like an addition to this software, or need help with your specific application, then we may be able to help. However, nothing in this life is for free, so we do invite you to sponsor this project, if you would like an addition or change made to it.
We can supply source code if required, under NDA, please contact us for more information.